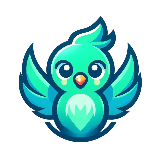
Java simple NetBeans application - URGENT
- or -
Post a project like this4480
£70(approx. $94)
- Posted:
- Proposals: 5
- Remote
- #225445
- Completed
Description
Experience Level: Intermediate
I need a simple JAVA program. The exact instructions (below) need to be followed. I need this job completed ASAP, during THIS weekend, and the latest at the end of this Monday (11th March).
I'll provide several .java files. Only 2 of these files need to be modified, and the work specifically relates to data structures and (traversal) sorting algorithms (pursudo code will be provided).
The instructions are as follows:
1: Create a class MyStringStack that implements the StringStack interface. Hint: you can store the data in an ArrayList of Strings.
2: Create a class MyStringQueue that implements the StringQueue interface. You can also use an ArrayList of Strings for this exercise.
3: Create a class MyNetwork that implements the Network interface.
4: Complete implementation of the Network Reader class (skelaton provided) so that it reads from an InputStream containing data in the following very simple format ( )
5: Return an ArrayList containing all of the stations that can be reached from a given point, and these stations should be in a “breadth first” order.
In a breadth first traversal of a graph we visit all of the nodes that can be reached from a given start point by traversing one connection, then all those that can be reached by traversing two connections, and so on.
You should use the following iterative algorithm to create the list:
Let Q be a queue of Strings
Create an initially empty list of Strings, which we will call L
Add the start station to the back of Q
While Q is not empty BEGIN
Remove the String at the front of Q, call this String S
Add S to the end of L
For each station S2 that is adjacent to S BEGIN
If S2 is not in the list L then add it to the back of queue Q
END (For)
END (While)
Return L
Clarification: in the algorithm two stations are “adjacent” if that you can get from one station to the other without passing through any other station. (I will give more explanation if you need it)
Note: Needs to NOT use recursive algorithm for breadth first traversal. Iterative algorithm only.
6: Implement the depthFirst method of the contained in the provided Navigator class. This method should return an ArrayList containing all of the stations that can be reached from a given point, and these stations should be in a “depth first” order. In the following manner:
Begin at the start point, then visit a station S2 that is adjacent the start point, then we try to visit an unvisited a station S3 that is adjacent from S2, and so on. If we arrive on a node from which we can go nowhere (because there are no adjacent nodes that we have not already visited) then we “backtrack” to a node which we have already visited but which has adjacent nodes that we have not visited.
You should use the following iterative algorithm to create the list. You can use the MyStringStack class that was created in task 1.
Let S be a stack of Strings
Create an initially empty list of Strings, which we will call L
Push the start station onto the stack S
While S is not empty BEGIN
Pop the String at the top of the stack. Call this String S.
If S is not already in list L then add it to the back of that list.
For each station S2 that is adjacent to S BEGIN
If S2 is not in the list L then push it on to the top of the stack S
END (For)
END (While)
Return L
Again don't use recursion, only use an iterative algorithm as in the above pseudo code.
..That's all. I can provide the files that I already have + a small data file for you to work with, plus some more information to help you complete the work.
Again, its very important to follow the instructions exactly, and to complete this before Monday.
Hopefully someone who is familiar with Java can complete this within a few hours.
I'll provide several .java files. Only 2 of these files need to be modified, and the work specifically relates to data structures and (traversal) sorting algorithms (pursudo code will be provided).
The instructions are as follows:
1: Create a class MyStringStack that implements the StringStack interface. Hint: you can store the data in an ArrayList of Strings.
2: Create a class MyStringQueue that implements the StringQueue interface. You can also use an ArrayList of Strings for this exercise.
3: Create a class MyNetwork that implements the Network interface.
4: Complete implementation of the Network Reader class (skelaton provided) so that it reads from an InputStream containing data in the following very simple format ( )
5: Return an ArrayList containing all of the stations that can be reached from a given point, and these stations should be in a “breadth first” order.
In a breadth first traversal of a graph we visit all of the nodes that can be reached from a given start point by traversing one connection, then all those that can be reached by traversing two connections, and so on.
You should use the following iterative algorithm to create the list:
Let Q be a queue of Strings
Create an initially empty list of Strings, which we will call L
Add the start station to the back of Q
While Q is not empty BEGIN
Remove the String at the front of Q, call this String S
Add S to the end of L
For each station S2 that is adjacent to S BEGIN
If S2 is not in the list L then add it to the back of queue Q
END (For)
END (While)
Return L
Clarification: in the algorithm two stations are “adjacent” if that you can get from one station to the other without passing through any other station. (I will give more explanation if you need it)
Note: Needs to NOT use recursive algorithm for breadth first traversal. Iterative algorithm only.
6: Implement the depthFirst method of the contained in the provided Navigator class. This method should return an ArrayList containing all of the stations that can be reached from a given point, and these stations should be in a “depth first” order. In the following manner:
Begin at the start point, then visit a station S2 that is adjacent the start point, then we try to visit an unvisited a station S3 that is adjacent from S2, and so on. If we arrive on a node from which we can go nowhere (because there are no adjacent nodes that we have not already visited) then we “backtrack” to a node which we have already visited but which has adjacent nodes that we have not visited.
You should use the following iterative algorithm to create the list. You can use the MyStringStack class that was created in task 1.
Let S be a stack of Strings
Create an initially empty list of Strings, which we will call L
Push the start station onto the stack S
While S is not empty BEGIN
Pop the String at the top of the stack. Call this String S.
If S is not already in list L then add it to the back of that list.
For each station S2 that is adjacent to S BEGIN
If S2 is not in the list L then push it on to the top of the stack S
END (For)
END (While)
Return L
Again don't use recursion, only use an iterative algorithm as in the above pseudo code.
..That's all. I can provide the files that I already have + a small data file for you to work with, plus some more information to help you complete the work.
Again, its very important to follow the instructions exactly, and to complete this before Monday.
Hopefully someone who is familiar with Java can complete this within a few hours.

Daniel D.
100% (47)Projects Completed
43
Freelancers worked with
42
Projects awarded
47%
Last project
18 Jul 2023
United Kingdom
New Proposal
Login to your account and send a proposal now to get this project.
Log inClarification Board Ask a Question
-
There are no clarification messages.
We collect cookies to enable the proper functioning and security of our website, and to enhance your experience. By clicking on 'Accept All Cookies', you consent to the use of these cookies. You can change your 'Cookies Settings' at any time. For more information, please read ourCookie Policy
Cookie Settings
Accept All Cookies