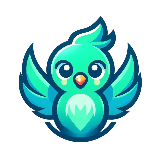
I need a Python programmer to pack and unpack a custom data type using MessagePack
- or -
Post a project like this$$
- Posted:
- Proposals: 2
- Remote
- #1062461
- Expired
Description
Experience Level: Intermediate
General information for the business: Inexperienced Python programmer
Kind of development: Customization of existing program
Description of requirements/functionality: I am trying to use MessagePack (http://msgpack.org/index.html) in Python 3 (msgpack-python) to pack an object of custom data type into bytes and then unpack this serialized object back into its original data type.
The data type is a MIMOSA DAReal, although, I don't think that this should have any effect on the method for packing/unpacking as there should just be a general method for dealing with custom data types in MessagePack.
On the MessagePack website at the bottom of the home page there are some examples of how to use the software in different programming languages. There is an example in the python section, labelled 'Packing/unpacking of custom data type':
import datetime
import msgpack
useful_dict = {
"id": 1,
"created": datetime.datetime.now(),
}
def decode_datetime(obj):
if b'__datetime__' in obj:
obj = datetime.datetime.strptime(obj["as_str"], "%Y%m%dT%H:%M:%S.%f")
return obj
def encode_datetime(obj):
if isinstance(obj, datetime.datetime):
return {'__datetime__': True, 'as_str': obj.strftime("%Y%m%dT%H:%M:%S.%f")}
return obj
packed_dict = msgpack.packb(useful_dict, default=encode_datetime)
this_dict_again = msgpack.unpackb(packed_dict, object_hook=decode_datetime)
This example takes a datetime object, serializes it into bytes and then deserializes it back into the same datetime object that it starts with. There is a (rather unhelpful) API for MessagePack python, that might help explain things like what default= and object_hook= do, http://pythonhosted.org/msgpack-python/api.html. I have attempted to use this example as a framework for my problem, but have been unsuccessful insofar.
MIMOSA is simply a collection of data types originally written as XSD files, I have converted these XSD files into Python classes and will send the script containing these classes when you need them.
The code I have attempted is as follows:
import msgpack
import sys
sys.path.append('/path/to/where/MIMOSA/classes/are/kept')
from OSACBM_all import DAReal #OSACBM_all is the file containing the MIMOSA classes
event = DAReal()
ID = int(5)
event.set_id(ID) #creates an OSACBM_all.DAReal object of type
useful_dict = {
"id": 1,
"event": event,
}
def decode_event(obj):
if b'DAReal' in obj:
obj = DAReal(obj[b'as_str'])
return obj
def encode_event(obj):
if isinstance(obj, DAReal):
return {'DAReal': True, 'as_str': str(obj)}
return obj
packed = msgpack.packb(useful_dict, default=encode_event)
unpacked = msgpack.unpackb(packed, object_hook=decode_event)
The packed part of this script seems to work (although I could be wrong) and produces a series of bytes of type .
However, the unpacked part is where I struggle. Although the returned event is indeed of the type , I believe that the script just creates a new DAReal event and discards the information stored in the original event that was packed. This is more clear when you try and extract the ID from the unpacked event:
ID = DARealEvent.get_id()
If you print this ID value it returns 'None', when a '5' was the expected value.
To summarize, I am looking for someone to write a script that can take a DAReal event, assign it a variable (such as ID), pack it into bytes, unpack it back into a DAReal event and extract the variable data.
Specific technologies required: Python
Extra notes: Don't hesitate to contact me if you need more information.
Kind of development: Customization of existing program
Description of requirements/functionality: I am trying to use MessagePack (http://msgpack.org/index.html) in Python 3 (msgpack-python) to pack an object of custom data type into bytes and then unpack this serialized object back into its original data type.
The data type is a MIMOSA DAReal, although, I don't think that this should have any effect on the method for packing/unpacking as there should just be a general method for dealing with custom data types in MessagePack.
On the MessagePack website at the bottom of the home page there are some examples of how to use the software in different programming languages. There is an example in the python section, labelled 'Packing/unpacking of custom data type':
import datetime
import msgpack
useful_dict = {
"id": 1,
"created": datetime.datetime.now(),
}
def decode_datetime(obj):
if b'__datetime__' in obj:
obj = datetime.datetime.strptime(obj["as_str"], "%Y%m%dT%H:%M:%S.%f")
return obj
def encode_datetime(obj):
if isinstance(obj, datetime.datetime):
return {'__datetime__': True, 'as_str': obj.strftime("%Y%m%dT%H:%M:%S.%f")}
return obj
packed_dict = msgpack.packb(useful_dict, default=encode_datetime)
this_dict_again = msgpack.unpackb(packed_dict, object_hook=decode_datetime)
This example takes a datetime object, serializes it into bytes and then deserializes it back into the same datetime object that it starts with. There is a (rather unhelpful) API for MessagePack python, that might help explain things like what default= and object_hook= do, http://pythonhosted.org/msgpack-python/api.html. I have attempted to use this example as a framework for my problem, but have been unsuccessful insofar.
MIMOSA is simply a collection of data types originally written as XSD files, I have converted these XSD files into Python classes and will send the script containing these classes when you need them.
The code I have attempted is as follows:
import msgpack
import sys
sys.path.append('/path/to/where/MIMOSA/classes/are/kept')
from OSACBM_all import DAReal #OSACBM_all is the file containing the MIMOSA classes
event = DAReal()
ID = int(5)
event.set_id(ID) #creates an OSACBM_all.DAReal object of type
useful_dict = {
"id": 1,
"event": event,
}
def decode_event(obj):
if b'DAReal' in obj:
obj = DAReal(obj[b'as_str'])
return obj
def encode_event(obj):
if isinstance(obj, DAReal):
return {'DAReal': True, 'as_str': str(obj)}
return obj
packed = msgpack.packb(useful_dict, default=encode_event)
unpacked = msgpack.unpackb(packed, object_hook=decode_event)
The packed part of this script seems to work (although I could be wrong) and produces a series of bytes of type .
However, the unpacked part is where I struggle. Although the returned event is indeed of the type , I believe that the script just creates a new DAReal event and discards the information stored in the original event that was packed. This is more clear when you try and extract the ID from the unpacked event:
ID = DARealEvent.get_id()
If you print this ID value it returns 'None', when a '5' was the expected value.
To summarize, I am looking for someone to write a script that can take a DAReal event, assign it a variable (such as ID), pack it into bytes, unpack it back into a DAReal event and extract the variable data.
Specific technologies required: Python
Extra notes: Don't hesitate to contact me if you need more information.

Ciaran B.
0% (0)Projects Completed
-
Freelancers worked with
-
Projects awarded
0%
Last project
13 Jul 2025
United Kingdom
New Proposal
Login to your account and send a proposal now to get this project.
Log inClarification Board Ask a Question
-
There are no clarification messages.
We collect cookies to enable the proper functioning and security of our website, and to enhance your experience. By clicking on 'Accept All Cookies', you consent to the use of these cookies. You can change your 'Cookies Settings' at any time. For more information, please read ourCookie Policy
Cookie Settings
Accept All Cookies