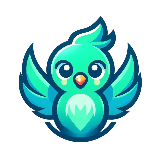
Help with back end programming on WIX
- or -
Post a project like this£28/hr(approx. $37/hr)
- Posted:
- Proposals: 9
- Remote
- #2782064
- Expired
Ranked Number-1 Service Providers on PPH: Expert IOS ANDROID Mobile App Developer | Web Developer |Digital Marketing|3D Animater

Top rated PHP Web Development | WordPress | Magento | Drupal | OpenCart | PrestaShop | Joomla

software engineer|Full-Stack Software Developer| web developer| App developer | AI Engineer | WordPress
102240838309321717607105075434271712841151385941926878383815852





Description
Experience Level: Expert
I have already done the majority of the work. However I now need someone to fine tune it and run me through what it all means.
I need a programmer who has experience with using the back end of WIX.
The project involves generating random questions from databases.
This is the code so far:
// For full API documentation, including code examples, visit http://wix.to/94BuAAs
import wixData from 'wix-data';
// Helper function to setup the table
function setupTable(showAnswers = false, resetData = false) {
// By default we always want to show the question column
var cols = [
{
"id": "col1",
"dataPath": "question",
"label": "Question",
"type": "string",
}
];
if(showAnswers) {
// Push (add) the answer column to the list of columns if we are viewing answers
cols.push(
{
"id": "col2",
"dataPath": "answerText",
"label": "Answer",
"type": "string",
},
);
}
// Set the columns on the table itself to be our array
$w('#resultsTable').columns = cols;
if(resetData) {
// Reset the tables data back to empty ([]) if we are reseting the data
$w('#resultsTable').rows = [];
}
}
function setupDropdown() {
// Query the Test dataset for values
wixData.query('Test')
.limit(1000) // Get all values
.ascending('title') // Sort by title ascending
.find()
.then(res => {
const uniqueTitles = getUniqueTitles(res.items); // Create a list of unique titles only
let cleanList = buildOptions(uniqueTitles); // Build them in to a format dropdown understands
$w("#topicFilter").options = cleanList; // Set the dropdown to be these values
$w("#topicFilter1").options = cleanList; // Set the dropdown to be these values
$w("#topicFilter2").options = cleanList; // Set the dropdown to be these values
})
// Converts a list of items with duplicates to a list of unique items
function getUniqueTitles(items){
const titlesOnly = items.map(item => item.title);
return [...new Set(titlesOnly)];
}
// Converts a list to objects (for use in dropdown)
function buildOptions(uniqueList) {
return uniqueList.map(curr => {
return { label: curr, value: curr };
});
}
}
// Run the filter to update the table
function processFilter(filterValue) {
var promise = wixData.query('EnterpriseDB')
.limit(1000) // Get all the values - we need to do this first
.contains('title', filterValue) // Filter based on the dropdown value
.find();
return promise;
}
function runFilter() {
var dropdownFilter = processFilter($w('#topicFilter').value);
var dropdownFilter1 = processFilter($w('#topicFilter1').value);
var dropdownFilter2 = processFilter($w('#topicFilter1').value);
Promise.all([dropdownFilter, dropdownFilter1, dropdownFilter2]).then(res => {
// Copy the results and randomise them
var results = shuffle(res[0].items.slice(0)).slice(0, $w('#topicSlider').value);
var results1 = shuffle(res[1].items.slice(0)).slice(0, $w('#topicSlider1').value);
var results2 = shuffle(res[2].items.slice(0)).slice(0, $w('#topicSlider2').value);
var outputResults = results.concat(results1).concat(results2);
// Set the results table rows to be the results
$w('#resultsTable').rows = outputResults;
});
// Helper function to shuffle
function shuffle(array) {
return array.sort(() => Math.random() - 0.5);
}
}
// Called on page load
$w.onReady(function () {
// Call setup table, don't show answers, do reset data
setupTable(false, true);
// Call setup dropdown
setupDropdown();
});
// Event for when dropdown is changed
export function topicFilter_change(event) {
runFilter();
}
// Event for when answers switch is changed
export function answersSwitch_change(event) {
setupTable($w('#answersSwitch').checked);
}
// Event for when slider changes
export function topicSlider_change(event) {
runFilter();
}
See the photo attached.
I need a programmer who has experience with using the back end of WIX.
The project involves generating random questions from databases.
This is the code so far:
// For full API documentation, including code examples, visit http://wix.to/94BuAAs
import wixData from 'wix-data';
// Helper function to setup the table
function setupTable(showAnswers = false, resetData = false) {
// By default we always want to show the question column
var cols = [
{
"id": "col1",
"dataPath": "question",
"label": "Question",
"type": "string",
}
];
if(showAnswers) {
// Push (add) the answer column to the list of columns if we are viewing answers
cols.push(
{
"id": "col2",
"dataPath": "answerText",
"label": "Answer",
"type": "string",
},
);
}
// Set the columns on the table itself to be our array
$w('#resultsTable').columns = cols;
if(resetData) {
// Reset the tables data back to empty ([]) if we are reseting the data
$w('#resultsTable').rows = [];
}
}
function setupDropdown() {
// Query the Test dataset for values
wixData.query('Test')
.limit(1000) // Get all values
.ascending('title') // Sort by title ascending
.find()
.then(res => {
const uniqueTitles = getUniqueTitles(res.items); // Create a list of unique titles only
let cleanList = buildOptions(uniqueTitles); // Build them in to a format dropdown understands
$w("#topicFilter").options = cleanList; // Set the dropdown to be these values
$w("#topicFilter1").options = cleanList; // Set the dropdown to be these values
$w("#topicFilter2").options = cleanList; // Set the dropdown to be these values
})
// Converts a list of items with duplicates to a list of unique items
function getUniqueTitles(items){
const titlesOnly = items.map(item => item.title);
return [...new Set(titlesOnly)];
}
// Converts a list to objects (for use in dropdown)
function buildOptions(uniqueList) {
return uniqueList.map(curr => {
return { label: curr, value: curr };
});
}
}
// Run the filter to update the table
function processFilter(filterValue) {
var promise = wixData.query('EnterpriseDB')
.limit(1000) // Get all the values - we need to do this first
.contains('title', filterValue) // Filter based on the dropdown value
.find();
return promise;
}
function runFilter() {
var dropdownFilter = processFilter($w('#topicFilter').value);
var dropdownFilter1 = processFilter($w('#topicFilter1').value);
var dropdownFilter2 = processFilter($w('#topicFilter1').value);
Promise.all([dropdownFilter, dropdownFilter1, dropdownFilter2]).then(res => {
// Copy the results and randomise them
var results = shuffle(res[0].items.slice(0)).slice(0, $w('#topicSlider').value);
var results1 = shuffle(res[1].items.slice(0)).slice(0, $w('#topicSlider1').value);
var results2 = shuffle(res[2].items.slice(0)).slice(0, $w('#topicSlider2').value);
var outputResults = results.concat(results1).concat(results2);
// Set the results table rows to be the results
$w('#resultsTable').rows = outputResults;
});
// Helper function to shuffle
function shuffle(array) {
return array.sort(() => Math.random() - 0.5);
}
}
// Called on page load
$w.onReady(function () {
// Call setup table, don't show answers, do reset data
setupTable(false, true);
// Call setup dropdown
setupDropdown();
});
// Event for when dropdown is changed
export function topicFilter_change(event) {
runFilter();
}
// Event for when answers switch is changed
export function answersSwitch_change(event) {
setupTable($w('#answersSwitch').checked);
}
// Event for when slider changes
export function topicSlider_change(event) {
runFilter();
}
See the photo attached.

Patrick F.
100% (3)Projects Completed
2
Freelancers worked with
2
Projects awarded
33%
Last project
17 Apr 2020
United Kingdom
New Proposal
Login to your account and send a proposal now to get this project.
Log inClarification Board Ask a Question
-
There are no clarification messages.
We collect cookies to enable the proper functioning and security of our website, and to enhance your experience. By clicking on 'Accept All Cookies', you consent to the use of these cookies. You can change your 'Cookies Settings' at any time. For more information, please read ourCookie Policy
Cookie Settings
Accept All Cookies