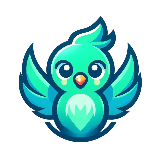
Create a simple, but beautifully written, PHP Command Line Interface (CLI) Program
- or -
Post a project like this- Posted:
- Proposals: 6
- Remote
- #1631813
- Awarded










Description
Kind of development: New program from scratch
Description of every module: A CLI PHP program that can run on Mac, PC or Linux. I am a PHP coder who does not have time to build all the tools I need. If you build the basic framework of the program, I can then refactor the script to add my specific domain knowledge.
Description of requirements/functionality: Let's exercise your PHP CLI programming skills.
I hope you might be able to create a program that performs a relatively simple function but which handles errors well and is well tested:
# XmlFileScanner
As a writer of XML files, I would like to be able to use a CLI PHP program to scan a given directory (targetDirectory) on PC or Mac and, for each file found with the `.xml` filename extension, set the following details into a variable; full path to file, relative path from the targetDirectory given, filename and filesize. Then, set those details as items in a variable which can be iterated by later code.
In addition to the arguments passed to the CLI program. The program should also read from an `ignored.txt` file which will reside in the same directory as the PHP script that was run. That file will contain a list of directory names (one per line) that should be ignored when the script is run when the 'recursive' option is TRUE.
# Input
The arguments passed to the CLI PHP program are:
1. `targetDirectory` (Mandatory). The path to the directory which should be scanned
2. `-r` (Optional). By default the script will just scan the files in the `targetDirectory`, if `-r` is passed as an argument, then sub-directories of the `targetDirectory` will also be scanned recursively.
The CLI Program should report errors to the Standard Error Stream:
* Fatal Error: Missing arguments. (If the `targetDirectory` is not provided)
* Fatal Error: Unknown directory(If the `targetDirectory` cannot be found)
* Fatal Error: Missing `ignored.txt` not found. (If the file containing directories to ignore is not found)
* Other errors that you think might be helpful.
# Output
Write output to Standard Output Stream.
* The total number of files found.
* The total number of Xml files found.
* The paths of directories that were skipped because they were in the list of ignored directories.
* The relative paths of Xml files found.
# Technologies
* Ideally you will use Object Oriented Programming where needed.
* Ideally you will make use of classes in the Standard PHP Library (SPL) (http://php.net/manual/en/book.spl.php) where possible.
* Ideally you will use Test Driven Development and provide PHPUnit Tests along with the code.
* Ideally you will provide some basic usage instructions along with the application.
I would be interested to hear your thoughts on how much this would cost me.
Specific technologies required: PHP OOP
OS requirements: Windows, Mac OS, Linux
Extra notes:
## Update: The following points have been added in response to discussions with Sellers ##
* The key point is that I am looking for a CLI application rather than a web application.
* Experience: You will have had previous experience in building CLI Applications in OOP PHP.
* If the code is written in OOP and it is written and structured well (i.e using patterns from Domain Driven Design, PofEAA, Gang of Four) then I find it easier to maintain.
* You could think of this task as a CLI Project Set-up (including Unit Tests) with slightly more than a 'Hello World' functionality. So, I understand that will add to the price. However, coders who have written a few OOP CLI PHP applications may well be able to base this on previously written OOP CLIP PHP applications which would reduce their costs.
* With regards to the version of PHP.
The PHP version must be no greater than PHP 7.* and no greater than PHP's current Current Stable release.
It would be easier for me if it was backward compatible with PHP 5.6 (or whichever PHP version comes pre-installed on Mac Sierra 10.12.5). But, that would be a bonus.
The 'model' aspect of the code could be re-used in a Drupal 8 custom module to be developed within 6 months. Drupal 8 requires PHP 5.6 ≤ ≥ 7 (https://www.drupal.org/docs/7/system-requirements/php#php_required)
Given those parameters, I would appreciate if it was 'backward compatible' as possible whilst making the best use of PHP's SPL (some very useful classes have been added since PHP 5.6).
So, in summary, it could be written in anything between: PHP 5.6 ≤ ≥ PHP 7.* (stable release)

John W.
100% (3)New Proposal
Login to your account and send a proposal now to get this project.
Log inClarification Board Ask a Question
-
Thanks for the quick reply. I was thinking about the error reporting. In Linux the apache error is reported to error_log file and its different for mac and windows. Is anything i'm missing in this case?
John W.06 Jul 2017Hi Sasikala,
I think the key point is that I am looking for a CLI application rather than a web application. So, there is no Apache involved.
See: https://stackoverflow.com/questions/9315714/what-is-difference-between-php-cli-and-php-cgi
and
http://php.net/manual/en/features.commandline.introduction.php
Some examples of CLI applications that have been written in PHP include:
Composer: https://getcomposer.org/download/
Drush: http://www.drush.org/
-
In the php script we need to redirect the error to the standard error output, So for windows, linux and mac we need to configure is as differently, right?
John W.06 Jul 2017Hi,
PHP executable is installed on each platform and does the parsing of the script. So, as long as the code is written in a platform independent way, then it should not need to be configured separately for each environment.
See: http://alvinalexander.com/php/php-write-stderr-php-command-line-script-fwrite
and see: https://stackoverflow.com/questions/6654157/how-to-get-a-platform-independent-directory-separator-in-php
Sasikala P.06 Jul 2017Yeah, I agree with that. it should write in to the device mapped as standard error. if its console then it will display the output there. Now its clear. I think I can do it now :)